データサイエンティストの必須知識、「オートエンコーダ | ディープラーニングの基礎」について解説します。
オートエンコーダの基礎
定義
オートエンコーダは、ニューラルネットワークの一種で、入力データを圧縮して再構築することを目的としています。具体的には、オートエンコーダは入力データを低次元の隠れ表現にエンコードし、その隠れ表現から元の入力データをデコードするように訓練されます。
主な用途
- 次元削減: データの主な特徴を捉えつつ、データの次元を減少できます。
- ノイズ除去: ノイズのある入力データからノイズを除去して、元のクリーンなデータを再構築します。
- 特徴抽出: 高次元のデータから重要な特徴を抽出するのに役立ちます。
オートエンコーダの仕組み
エンコーダ
エンコーダは、オートエンコーダの前半部分であり、入力データを低次元の隠れ表現に変換します。
入力データの圧縮
エンコーダは、高次元の入力データを低次元の隠れ表現に圧縮します。これは、データの主な特徴を維持しつつ、データ量を削減するためのものです。
数式で表すと、エンコーダ関数は以下のようになります:
\[
h = f(x)
\]
ここで、\( x \) は入力データ、\( h \) は隠れ表現、そして \( f \) はエンコーダ関数です。
隠れ層への変換
隠れ表現 \( h \) は、エンコーダによって生成された低次元のデータです。この隠れ表現は、デコーダによって元の入力データに再変換されます。
Pythonの疑似コードでのエンコーダの例:
import tensorflow as tf
# Define the encoder
class Encoder(tf.keras.layers.Layer):
def __init__(self, intermediate_dim):
super(Encoder, self).__init__()
self.hidden_layer = tf.keras.layers.Dense(units=intermediate_dim, activation=tf.nn.relu)
def call(self, input_features):
activation = self.hidden_layer(input_features)
return activation
デコーダ
デコーダはオートエンコーダの後半部分を担当し、エンコーダによって圧縮された隠れ表現を元の入力データに再変換します。
圧縮データの復元
デコーダは隠れ表現から元のデータの次元にデータを復元します。これは、エンコーダが捉えたデータの主な特徴をもとに、元のデータを再構築するプロセスです。
元の入力への変換
デコーダの目的は、エンコーダによって生成された隠れ表現を使用して、元の入力データにできるだけ近いデータを再構築することです。
Pythonの疑似コードでのデコーダの例:
import tensorflow as tf
# Define the decoder
class Decoder(tf.keras.layers.Layer):
def __init__(self, original_dim):
super(Decoder, self).__init__()
self.output_layer = tf.keras.layers.Dense(units=original_dim, activation=tf.nn.sigmoid)
def call(self, encoded):
activation = self.output_layer(encoded)
return activation
学習の仕組み
損失関数
オートエンコーダの学習の際には、損失関数として「再構築エラー」を使用します。これは、再構築されたデータと元の入力データとの間の差異を計測するものです。
再構築エラー
再構築エラーは、オリジナルのデータ \( x \) と再構築されたデータ \( \hat{x} \) との差を示します。一般的には平均二乗誤差 (MSE) が用いられます。
\[
\text{MSE} = \frac{1}{n} \sum_{i=1}^{n} (x_i – \hat{x}_i)^2
\]
最適化手法
最適化手法は、損失関数を最小化するための手法です。一般的には、AdamやSGDなどの最適化アルゴリズムがオートエンコーダの学習に使用されます。
Pythonの疑似コードでの最適化の例:
optimizer = tf.keras.optimizers.Adam(learning_rate=0.001)
loss_function = tf.keras.losses.MeanSquaredError()
# Training step
def train_step(model, input_data, loss_function, optimizer):
with tf.GradientTape() as tape:
reconstructed = model(input_data)
loss = loss_function(input_data, reconstructed)
gradients = tape.gradient(loss, model.trainable_variables)
optimizer.apply_gradients(zip(gradients, model.trainable_variables))
return loss
AIエンジニア
オートエンコーダの応用例
オートエンコーダは、その基本的な機能に加えて、多くの応用シーンで使用されます。以下に、主な応用例をいくつか紹介します。
ノイズ除去
オートエンコーダは、ノイズ除去のためにも使用できます。具体的には、ノイズのあるデータをオートエンコーダの入力として提供し、ノイズのないデータをターゲットとして学習させます。学習後のオートエンコーダは、ノイズのある新しいデータをクリーンに再構築できます。
Pythonの疑似コード例:
# Assuming autoencoder is a pre-defined autoencoder model
# Introduce noise to data
noisy_data = original_data + noise
# Train the autoencoder
autoencoder.fit(noisy_data, original_data)
# Use the autoencoder to denoise new data
denoised_data = autoencoder.predict(new_noisy_data)
次元削減
次元削減は、データの次元数を減らすことで、データを視覚化したり、計算コストを削減したりするための手法です。オートエンコーダの隠れ層の出力は、入力データの圧縮表現となるため、これを次元削減のために使用できます。
Pythonの疑似コード例:
# Assuming encoder is the encoder part of a pre-defined autoencoder model
# Transform data to a lower-dimensional space
compressed_data = encoder.predict(original_data)
異常検知
オートエンコーダは、異常検知にも使用されます。正常なデータのみを使用してオートエンコーダを訓練した後、新しいデータをオートエンコーダに入力して再構築します。再構築エラーが大きい場合、そのデータは異常であると判定されます。
Pythonの疑似コード例:
# Assuming autoencoder is a pre-defined autoencoder model
# Train the autoencoder with normal data
autoencoder.fit(normal_data, normal_data)
# Calculate reconstruction error for new data
reconstructed_data = autoencoder.predict(new_data)
reconstruction_error = mean_squared_error(new_data, reconstructed_data)
# If the error is above a threshold, the data is considered an anomaly
if reconstruction_error > threshold:
print("Anomaly detected!")
オートエンコーダの変種
オートエンコーダにはいくつかの変種が存在し、異なる目的や応用に応じて利用されます。
変分オートエンコーダ (VAE)
変分オートエンコーダ (VAE) は、オートエンコーダの一種で、確率的にエンコードとデコードします。VAEは、エンコーダが平均と標準偏差を出力し、これを使用して隠れ表現をサンプリングします。
数式での主な違いは、損失関数にKLダイバージェンスが追加される点です。これにより、学習中に隠れ表現が正規分布に近づくように制約されます。
疎オートエンコーダ
疎オートエンコーダは、隠れ層の活性化を制約して、一部のユニットのみがアクティブになるように設計されています。これにより、オートエンコーダがデータの重要な特徴を捉える能力が強化されます。
畳み込みオートエンコーダ
画像データなどのグリッド状のデータを処理する際に効果的なのが、畳み込みオートエンコーダです。このオートエンコーダは、畳み込み層を使用してデータをエンコードおよびデコードします。
実際の実装例
オートエンコーダの概念を理解した後、次に重要なのは、その知識を実際のコードに変換することです。以下に、シンプルなオートエンコーダの実装例と一部の応用例を紹介します。
シンプルなオートエンコーダの実装
PythonのKerasライブラリを使用して、シンプルなオートエンコーダを実装します。
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense
from tensorflow.keras.models import Model
# Define the autoencoder's architecture
input_layer = Input(shape=(784,))
encoded = Dense(128, activation='relu')(input_layer)
decoded = Dense(784, activation='sigmoid')(encoded)
autoencoder = Model(input_layer, decoded)
# Compile the model
autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
# Assuming x_train is the training data
autoencoder.fit(x_train, x_train, epochs=50, batch_size=256, shuffle=True)
応用例の実装
ノイズ除去オートエンコーダの簡単な例:
import numpy as np
# Adding noise to the data
noise_factor = 0.5
x_train_noisy = x_train + noise_factor * np.random.normal(loc=0.0, scale=1.0, size=x_train.shape)
# Clipping the values to be between 0 and 1
x_train_noisy = np.clip(x_train_noisy, 0., 1.)
# Train the autoencoder with noisy data
autoencoder.fit(x_train_noisy, x_train, epochs=100, batch_size=256, shuffle=True)
まとめ
オートエンコーダは、非監視学習の一形態であり、データの圧縮やノイズ除去、特徴抽出などの多岐にわたるタスクに使用されます。オートエンコーダの背後にある理論を理解し、実際のコードでそれを実装する能力は、データサイエンスや機械学習の領域で非常に価値があります。
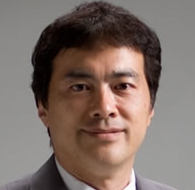
▼IT人材は2030年に国内で79万人, 全世界で「8,500万人」以上不足!
▼世界の平均年収はなんと「1,000万円」以上!
▼自宅 + パソコン + 無料翻訳ツールで「全世界が仕事場!」
AIエンジニア